Navigation은 무엇일까?
Navigation Architecture Component(이하 Navigation)는 18년도에 처음 소개된 Android Jetpack Library입니다.
Fragments, Activities와 같은 components 간 탐색을 돕기 위한 라이브러리입니다.
Android Jetpack의 Navigation 구성 요소는 간단한 버튼 클릭부터 앱 바 및 탐색 창과 같은 더 복잡한 패턴까지 탐색을 구현하는 데 도움이 됩니다.
single activity, multiple fragment 구조를 구현하기 쉽게 해 줍니다.
Navigation 사용하여 할 수 있는 것들
- Fragment 트랜잭션을 관리할 수 있다.
- Up, Back 버튼의 작업 등(백 스택 관리)을 간단하게 처리
- 화면 전환 시, Animation이나 Transition을 위한 표준화된 리소스를 제공
- 딥링크 구현 및 처리
- Navigation UI 패턴을 사용한 Navigation drawers, Bottom Navigation의 연동을 쉽게 구현 가능하게 지원
- fragment 간의 이동시 안전하게 데이터를 전달 가능
- Navigation Editor를 통해 화면 흐름을 시각적으로 관리할 수 있다.
기본 FragmentManager, Intent , Bundle 등의 사용을 대체할 수 있습니다.
Navigation의 구성요소
Navigation에는 세 가지 구성요소가 있습니다.
1. navigation graph
모든 내비게이션 관련된 정보를 가진 xml resource 파일입니다.
여기에는 대상(destinations)이라고 부르는 앱 내의 모든 개별적 콘텐츠 영역과 사용자가 앱에서 갈 수 있는 모든 이용 가능한 경로가 포함됩니다.
NavGraph는 XML 작성하거나 Visual Editor를 통해서 작업할 수 있습니다. 아래는 navigation XML에서 사용할 수 있는 기본 태그입니다. 참고로 Navigation XML은 res/navigation/ 폴더에 추가해주어야 합니다.
- <navigation> : NavGraph
- <fragment> or <activity> : NavDestination
- <action> : NavAction (handled in NavDirections)
- <argument> : NavArgs
아래는 Fragment 5개로 이루어진 NavGraph 예시입니다.
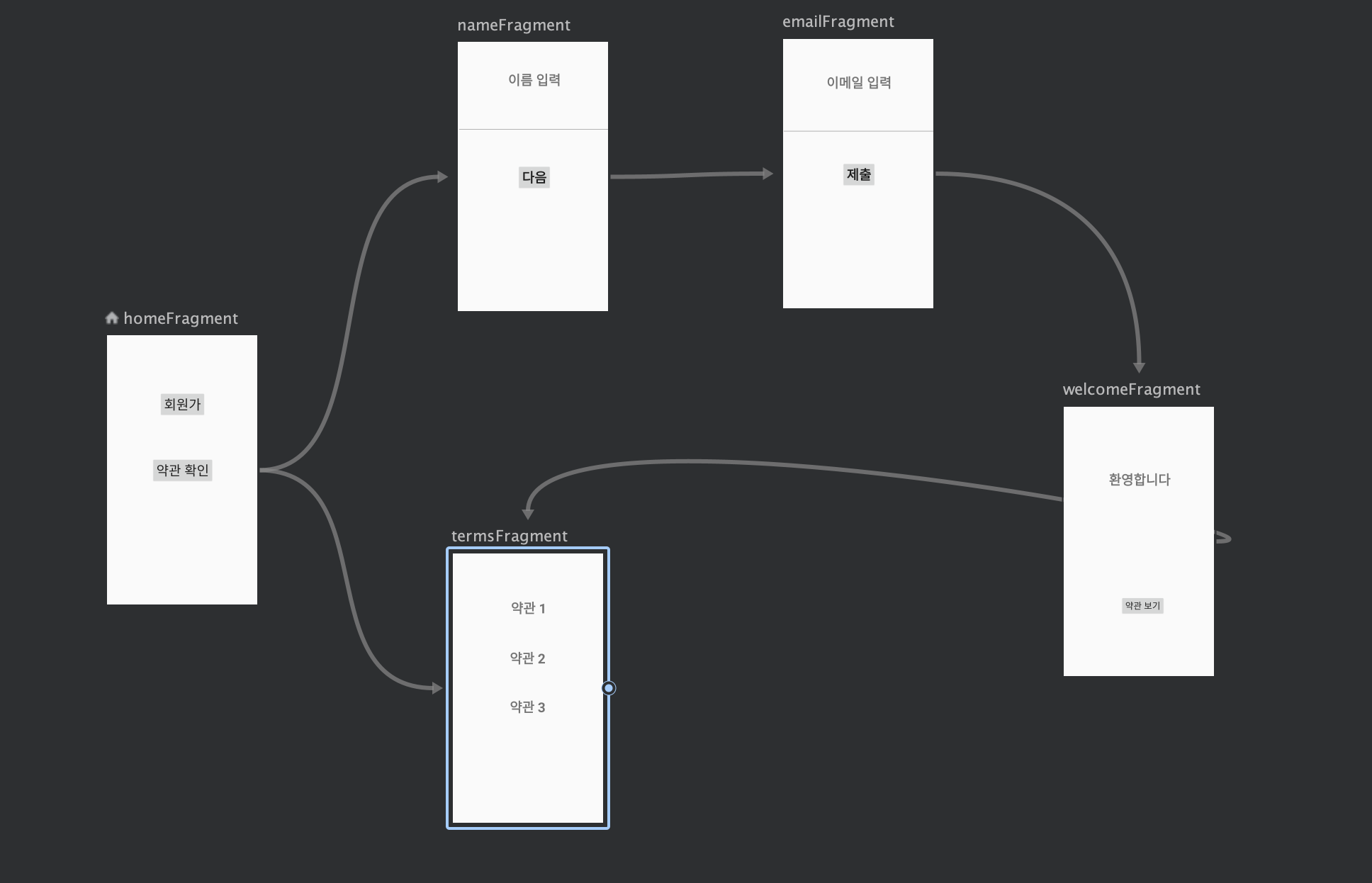
<?xml version="1.0" encoding="utf-8"?>
<navigation xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/nav_graph"
app:startDestination="@id/homeFragment">
<fragment
android:id="@+id/homeFragment"
android:name="com.keepseung.navdemo.HomeFragment"
android:label="HomeFragment"
tools:layout="@layout/fragment_home">
<action
android:id="@+id/action_homeFragment_to_nameFragment"
app:destination="@id/nameFragment"
app:enterAnim="@anim/slide_in_left"
app:exitAnim="@anim/slide_in_right"
app:popEnterAnim="@anim/slide_out_right"
app:popExitAnim="@anim/slide_out_left" />
<action
android:id="@+id/action_homeFragment_to_termsFragment"
app:destination="@id/termsFragment"
app:enterAnim="@anim/push_down_in"
app:exitAnim="@anim/push_down_out"
app:popEnterAnim="@anim/push_up_in"
app:popExitAnim="@anim/push_up_out" />
</fragment>
<fragment
android:id="@+id/emailFragment"
android:name="com.keepseung.navdemo.EmailFragment"
android:label="EmailFragment"
tools:layout="@layout/fragment_email"
>
<action
android:id="@+id/action_emailFragment_to_welcomeFragment"
app:destination="@id/welcomeFragment"
app:enterAnim="@anim/slide_in_left"
app:exitAnim="@anim/slide_in_right"
app:popEnterAnim="@anim/slide_out_right"
app:popExitAnim="@anim/slide_out_left" />
</fragment>
<fragment
android:id="@+id/welcomeFragment"
android:name="com.keepseung.navdemo.WelcomeFragment"
android:label="WelcomeFragment"
tools:layout="@layout/fragment_welcome"
>
<action
android:id="@+id/action_welcomeFragment_to_termsFragment"
app:destination="@id/termsFragment"
app:enterAnim="@anim/push_down_in"
app:exitAnim="@anim/push_down_out"
app:popEnterAnim="@anim/push_up_in"
app:popExitAnim="@anim/push_up_out" />
</fragment>
<fragment
android:id="@+id/termsFragment"
android:name="com.keepseung.navdemo.TermsFragment"
android:label="fragment_terms"
tools:layout="@layout/fragment_terms" />
<fragment
android:id="@+id/nameFragment"
android:name="com.keepseung.navdemo.NameFragment"
android:label="NameFragment"
tools:layout="@layout/fragment_name"
>
<action
android:id="@+id/action_nameFragment_to_emailFragment"
app:destination="@id/emailFragment"
app:enterAnim="@anim/slide_in_left"
app:exitAnim="@anim/slide_in_right"
app:popEnterAnim="@anim/slide_out_right"
app:popExitAnim="@anim/slide_out_left" />
</fragment>
</navigation>
각각의 Fragment는 하나의 화면인 Destination을 의미하고,
Fragment내에 action, argument태그는 각각 화면을 이동 , 파라미터를 의미합니다.
2. NavHost
navigation graph에서 대상(destinations)을 표시하는 빈 컨테이너입니다.
Navigation 구성요소에는 프래그먼트 대상(destinations)을 표시하는 기본 NavHost을 구현한 NavHostFragment가 포함됩니다.
예시로 activity_main.xml에 다음과 같이 추가할 수 있습니다.
다음 코드를 통해서 알고 있어야 할 부분은
1. NavHostFragment를 이름으로 가진 Fragement라는 것
2. default NavHost 것
3. navigation graph에 해당하는 xml 파일 경로 등이 있습니다.
<fragment
android:id="@+id/fragment"
android:name="androidx.navigation.fragment.NavHostFragment"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:defaultNavHost="true"
app:navGraph="@navigation/nav_graph"/>
3. NavController
NavHost에서 앱 navigation을 관리하는 객체입니다.
NavController는 사용자가 앱 내에서 이동할 때 NavHost에서 대상(destination) 콘텐츠의 전환을 처리합니다.
앱을 탐색하는 동안 탐색 그래프에서 특정 경로를 따라 이동할지, 특정 대상으로 직접 이동할지 NavController에게 전달합니다. 그러면 NavController가 NavHost에 적절한 대상을 표시합니다.
A Destination에서 B Destination으로 이동하는 것을 나타낸 구조입니다.
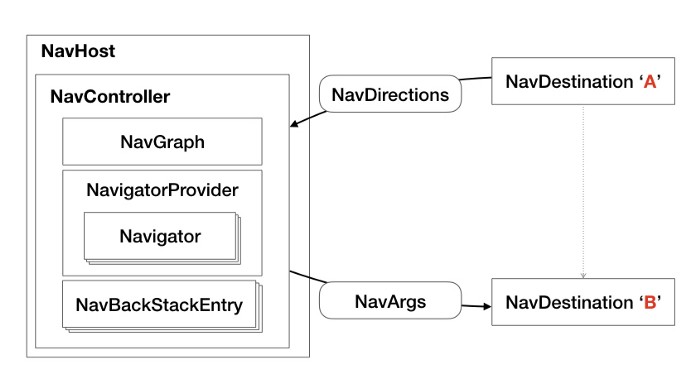
다음 글
- AAC Navigation 살펴보기
- AAC Navigation 사용하기 (이동, 데이터 전송, 애니메이션)
'Android > Jetpack, Clean Architecture' 카테고리의 다른 글
[Android] AAC Navigation 사용하기 (이동, 데이터 전송, 애니메이션) (0) | 2021.02.16 |
---|---|
Android 양방향 데이터 바인딩 사용하기 (Two-way DataBinding With LiveData) (0) | 2021.02.16 |
DataBinding에 ViewModel, LiveData와 함께 사용하기 (0) | 2021.02.16 |
[안드로이드/Android] LiveData 사용하기 (0) | 2021.01.25 |
[안드로이드/Android] ViewModel 사용하기 (0) | 2021.01.20 |